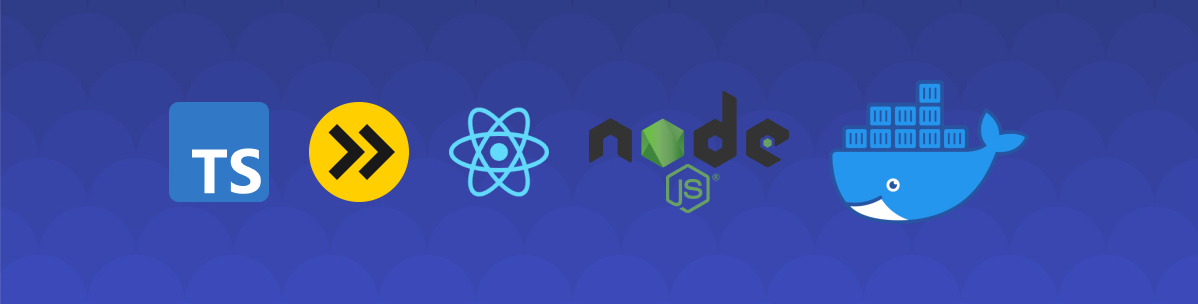
Creating a web application using Yarn workspace, TypeScript, esbuild, React, and Express — part 3
This article will guide you through setting up a basic web application using Yarn's workspace, TypeScript, esbuild, Express, and React. At the end of this tutorial you will have a fully buildable and deployable web application.
- Setting up the project — part 1
- Adding code — part 2
- Building the app — part 3
- Going further — advanced
Want to see the full code? Check out the repository on GitHub at halftheopposite/tutorial-app.
In this third and final part, we will implement the scripts used to build and launch our application.
Bundlers
To transpile our TypeScript code into interpretable JavaScript, and to bundle all external libraries into a single file, we will be using a bundler tool. Many bundlers are available in the JS/TS ecosystem, such as WebPack, Parcel, or Rollup, but the one that we will be choosing is esbuild. In comparison to others bundlers, esbuild
comes with many features loaded by default (TypeScript, React) and has huge performances gains (up to a hundred times faster). If you're interested in knowing more, please take a time to read this FAQ by the author.
The scripts will require the following dependencies:
From the project's root, run: yarn add -D -W esbuild ts-node
.
package.json
1{ 2 "name": "my-app", 3 "version": "1.0", 4 "license": "UNLICENSED", 5 "private": true, 6 "workspaces": ["packages/*"], 7 "devDependencies": { 8 "esbuild": "^0.9.6", 9 "ts-node": "^9.1.1", 10 "typescript": "^4.2.3" 11 }, 12 "scripts": { 13 "app": "yarn workspace @my-app/app", 14 "common": "yarn workspace @my-app/common", 15 "server": "yarn workspace @my-app/server" 16 } 17}
Build
We now have all the tools needed to build our application, so let's create our first script.
Start by creating a new folder named scripts/
at the project's root.
Our script will be written in TypeScript and executed with ts-node
from the command line. Although a CLI exists for esbuild
, it is more convenient to use the library through JS or TS if you want to pass more complex parameters or to combine multiple workflows together.
Create a build.ts
file inside the scripts/
folder and add the code below (I will explain what the code does through comments):
scripts/build.ts
1import { build } from 'esbuild'; 2 3/** 4 * Generic options passed during build. 5 */ 6interface BuildOptions { 7 env: 'production' | 'development'; 8} 9 10/** 11 * A builder function for the app package. 12 */ 13export async function buildApp(options: BuildOptions) { 14 const { env } = options; 15 16 await build({ 17 entryPoints: ['packages/app/src/index.tsx'], // We read the React application from this entrypoint 18 outfile: 'packages/app/public/script.js', // We output a single file in the public/ folder (remember that the "script.js" is used inside our HTML page) 19 define: { 20 'process.env.NODE_ENV': `"${env}"`, // We need to define the Node.js environment in which the app is built 21 }, 22 bundle: true, 23 minify: env === 'production', 24 sourcemap: env === 'development', 25 }); 26} 27 28/** 29 * A builder function for the server package. 30 */ 31export async function buildServer(options: BuildOptions) { 32 const { env } = options; 33 34 await build({ 35 entryPoints: ['packages/server/src/index.ts'], 36 outfile: 'packages/server/dist/index.js', 37 define: { 38 'process.env.NODE_ENV': `"${env}"`, 39 }, 40 external: ['express'], // Some libraries have to be marked as external 41 platform: 'node', // When building for node we need to setup the environement for it 42 target: 'node14.15.5', 43 bundle: true, 44 minify: env === 'production', 45 sourcemap: env === 'development', 46 }); 47} 48 49/** 50 * A builder function for all packages. 51 */ 52async function buildAll() { 53 await Promise.all([ 54 buildApp({ 55 env: 'production', 56 }), 57 buildServer({ 58 env: 'production', 59 }), 60 ]); 61} 62 63// This method is executed when we run the script from the terminal with ts-node 64buildAll();
The code is pretty self-explanatory, but if you feel that you're missing pieces you can have a look at esbuild
's API documentation to have a complete list of keywords.
Our build script is now complete! The last thing we need to do is to add a new command into our package.json
to conveniently run the build operations.
1{ 2 "name": "my-app", 3 "version": "1.0", 4 "license": "UNLICENSED", 5 "private": true, 6 "workspaces": ["packages/*"], 7 "devDependencies": { 8 "esbuild": "^0.9.6", 9 "ts-node": "^9.1.1", 10 "typescript": "^4.2.3" 11 }, 12 "scripts": { 13 "app": "yarn workspace @my-app/app", 14 "common": "yarn workspace @my-app/common", 15 "server": "yarn workspace @my-app/server", 16 "build": "ts-node ./scripts/build.ts" // Add this line here 17 } 18}
We can now run yarn build
from the project's root folder to launch the build process everytime you make changes to your project (we will see how we can add hot-reloading in another post).
Structure reminder:
1my-app/ 2├─ packages/ 3├─ scripts/ 4│ ├─ build.ts 5├─ package.json 6├─ tsconfig.json
Serve
Our application is built and ready to be served to the world, and we just need to add a last command to our package.json
:
1{ 2 "name": "my-app", 3 "version": "1.0", 4 "license": "UNLICENSED", 5 "private": true, 6 "workspaces": ["packages/*"], 7 "devDependencies": { 8 "esbuild": "^0.9.6", 9 "ts-node": "^9.1.1", 10 "typescript": "^4.2.3" 11 }, 12 "scripts": { 13 "app": "yarn workspace @my-app/app", 14 "common": "yarn workspace @my-app/common", 15 "server": "yarn workspace @my-app/server", 16 "build": "ts-node ./scripts/build.ts", 17 "serve": "node ./packages/server/dist/index.js" // Add this line here 18 } 19}
Since we are now dealing with pure JavaScript we can use the node
binary to launch our server. So go on and run yarn serve
.
If you look at the console you'll see that the server is successfully listening. You can also open a web browser and navigate to http://localhost:3000 to display your functional React application 🎉!
If you'd like to change the port at runtime, you can launch the serve
command by prefixing it with an environment variable: PORT=4000 yarn serve
.
Summary
If you've read this far, then congratulations on completing your modern web application! If you still have questions or feedback on this serie of articles, please do not hesitate to reach out.
What's next?
Some things that we will explore in an appendix post:
- Creating a containerized application with Docker
- Adding CI with GitHub Actions
- Deploying our container to Heroku
- Adding a hot-reloading mode with
esbuild
Posted on March 13, 2021